Learning React: Functional Web Development with React and Redux
This book is for developers who want to learn the React library while learning the different way of thinking about and approaching web development.
Learning React
Boston. Learning React. Functional Web Development with React and. Redux O'Reilly books may be purchased for educational business
Learning React Functional Web Development With React And Redux
learning-react-functional-web-development-with-react-and-redux. 1/1. Downloaded from uniport.edu.ng on. September 17 2022 by guest.
WEB APPLICATION DEVELOPMENT
Alex Banks and Eve Porcello “Learning React: Functional Web Development with React and Redux”
STELLA MARIS COLLEGE (AUTONOMOUS) CHENNAI Course
07-Sept-2022 Lecture /. Demo. Banks Alex
Developing a frontend application using ReactJS and Redux
the entire development process. Keywords: Web application development ReactJS
React JS – An Emerging Frontend Javascript Library
MVC model Web application development. development for developers to design rich UI's. React ... [8] “Learning React Functional Web Development.
SCHEME & SYLLABUS OF POSTGRADUATE DEGREE COURSE
01-Nov-2021 MCA-BC-110: Computer Fundamentals and Programming in C. Credit: 3 ... “Learning React: Functional Web Development with React and Redux”.
Become a Web Developer
04. Functional Programming. 05. Asynchronous Programming in JavaScript. 06. Master the React ecosystem with Redux and Jest. 07. Web APIs and Asynchronous
French ICUs fight back: An example of regional ICU organisation to
Learning React: functional web development with React and. Redux. Sebastopol: O'Reilly Media; 2017. 5.Covid-19. Fiches pratiques. https://covid.com-scape.fr/;
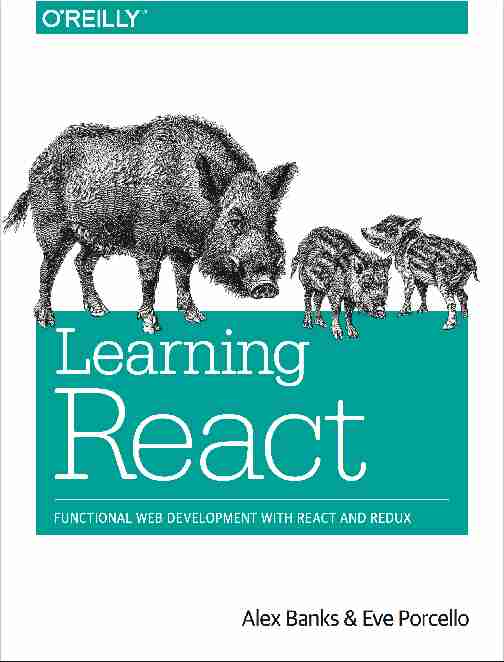
Alex Banks and Eve Porcello
Learning React
Functional Web Development
with React and Redux BostonFarnhamSebastopolTokyoBeijingBostonFarnhamSebastopolTokyoBeijing978-1-491-95462-1
[LSI]Learning React by Alex Banks and Eve Porcello Copyright © 2017 Alex Banks and Eve Porcello. All rights reserved.Printed in the United States of America.
Published by O'Reilly Media, Inc., 1005 Gravenstein Highway North, Sebastopol, CA 95472.O'Reilly books may be purchased for educational, business, or sales promotional use. Online editions are
also available for most titles (http://oreilly.com/safari). For more information, contact our corporate/insti-
tutional sales department: 800-998-9938 or corporate@oreilly.com.Editor: Allyson MacDonaldProduction Editor: Melanie Yarbrough
Copyeditor: Colleen Toporek
Proofreader: Rachel HeadIndexer: WordCo Indexing ServicesInterior Designer: David Futato
Cover Designer: Karen Montgomery
Illustrator: Rebecca DemarestMay 2017:
First Edition
Revision History for the First Edition
2017-04-26: First Release
See http://oreilly.com/catalog/errata.csp?isbn=9781491954621 for release details.The O'Reilly logo is a registered trademark of O'Reilly Media, Inc. Learning React, the cover image, and
related trade dress are trademarks of O'Reilly Media, Inc. While the publisher and the authors have used good faith efforts to ensure that the information andinstructions contained in this work are accurate, the publisher and the authors disclaim all responsibility
for errors or omissions, including without limitation responsibility for damages resulting from the use of
or reliance on this work. Use of the information and instructions contained in this work is at your own
risk. If any code samples or other technology this work contains or describes is subject to open source
licenses or the intellectual property rights of others, it is your responsibility to ensure that your use
thereof complies with such licenses and ghts.Table of Contents
Preface. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. . . . . . . . . . ix 1.Welcome to React. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. . . 1Obstacles and Roadblocks 1
React Is a Library 2
New ECMAScript Syntax 2
Popularity of Functional JavaScript 2
JavaScript Tooling Fatigue 2
Why React Doesn't Have to Be Hard to Learn 3
React's Future 3
Keeping Up with the Changes 4
Working with the Files 4
File Repository 4
React Developer Tools 5
Installing Node.js 6
2.Emerging JavaScript. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. . 9Declaring Variables in ES6 10
const 10 let 10Template Strings 12
Default Parameters 13
Arrow Functions 14
Transpiling ES6 17
ES6 Objects and Arrays 19
Destructuring Assignment 19
Object Literal Enhancement 20
The Spread Operator 22
iiiPromises
24Classes
25ES6 Modules 27
CommonJS 283.
Functional Programming with JavaScript. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31
What It Means to Be Functional 32
Imperative Versus Declarative 34
Functional Concepts 36
Immutability 36
Pure Functions 38
Data Transformations 41
Higher-Order Functions 48
Recursion 49
Composition 52
Putting It All Together 54
4.Pure React. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. . . . . . 59Page Setup
59The Virtual DOM 60
React Elements 62
ReactDOM 64
Children
65Constructing Elements with Data 67
React Components 68
React.createClass 69
React.Component 72
Stateless Functional Components 73
DOM Rendering 74
Factories
775.
React with JSX. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. . . . 81React Elements as JSX 81
JSX Tips 82
Babel 84Recipes as JSX
85Intro to Webpack 93
Webpack Loaders 94
Recipes App with a Webpack Build 94
6.Props, State, and the Component Tree. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 109
Property Validation 109
iv | Table of ContentsValidating Props with createClass 110
Default Props 114
Custom Property Validation 115
ES6 Classes and Stateless Functional Components 116 Refs 119Inverse Data Flow 121
Refs in Stateless Functional Components 123
React State Management 123
Introducing Component State 124
Initializing State from Properties 128
State Within the Component Tree 130
Color Organizer App Overview 130
Passing Properties Down the Component Tree 131Passing Data Back Up the Component Tree 1347.
Enhancing Components. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141
Component Lifecycles 141
Mounting Lifecycle 142
quotesdbs_dbs2.pdfusesText_2[PDF] learning specialist job description
[PDF] learning techniques
[PDF] learning techniques for adults
[PDF] learning theories and their application to classroom pdf
[PDF] learning theories and their application to classroom ppt
[PDF] learning theories pdf
[PDF] learning welsh
[PDF] learning react 2nd edition github
[PDF] lease of a dwelling québec
[PDF] leased departments examples
[PDF] leasing land to cell phone companies
[PDF] leather industry
[PDF] lebanese civil code
[PDF] lebanese court system